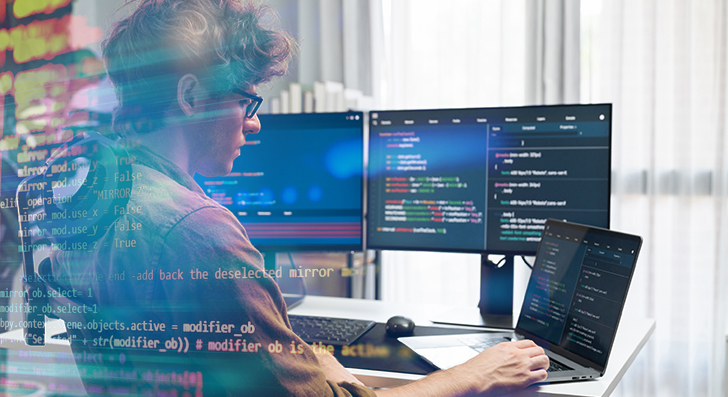
Scalability indicates your software can take care of progress—much more users, additional knowledge, and a lot more site visitors—with out breaking. To be a developer, constructing with scalability in mind will save time and anxiety afterwards. Listed here’s a clear and realistic guidebook that will help you get started by Gustavo Woltmann.
Design and style for Scalability from the beginning
Scalability isn't really some thing you bolt on afterwards—it should be portion of one's system from the beginning. Quite a few applications are unsuccessful once they improve quick mainly because the original style and design can’t deal with the additional load. As a developer, you must think early about how your process will behave under pressure.
Start off by designing your architecture for being adaptable. Steer clear of monolithic codebases the place everything is tightly connected. As a substitute, use modular design or microservices. These designs split your application into smaller, impartial sections. Each module or support can scale By itself without the need of affecting The entire technique.
Also, give thought to your database from day a single. Will it will need to take care of a million customers or perhaps 100? Pick the ideal type—relational or NoSQL—according to how your knowledge will increase. Approach for sharding, indexing, and backups early, Even when you don’t need them however.
Yet another critical place is to stay away from hardcoding assumptions. Don’t generate code that only works under present situations. Take into consideration what would come about if your user base doubled tomorrow. Would your application crash? Would the databases decelerate?
Use style and design designs that guidance scaling, like concept queues or function-pushed units. These assistance your application cope with additional requests devoid of receiving overloaded.
Whenever you Create with scalability in mind, you're not just preparing for fulfillment—you happen to be minimizing foreseeable future head aches. A effectively-planned system is less complicated to maintain, adapt, and develop. It’s better to prepare early than to rebuild afterwards.
Use the ideal Databases
Picking out the proper database is a essential Portion of developing scalable applications. Not all databases are crafted a similar, and utilizing the Incorrect you can sluggish you down and even lead to failures as your app grows.
Get started by comprehension your info. Can it be hugely structured, like rows within a desk? If Indeed, a relational database like PostgreSQL or MySQL is a superb in good shape. These are typically sturdy with relationships, transactions, and regularity. They also guidance scaling strategies like read replicas, indexing, and partitioning to manage much more website traffic and facts.
In case your details is much more adaptable—like user action logs, product catalogs, or paperwork—consider a NoSQL selection like MongoDB, Cassandra, or DynamoDB. NoSQL databases are better at managing big volumes of unstructured or semi-structured facts and can scale horizontally far more easily.
Also, take into account your browse and compose designs. Are you undertaking plenty of reads with less writes? Use caching and skim replicas. Are you dealing with a significant write load? Explore databases which will tackle higher publish throughput, or simply function-dependent details storage methods like Apache Kafka (for short term facts streams).
It’s also good to think ahead. You may not want State-of-the-art scaling attributes now, but selecting a database that supports them signifies you received’t have to have to modify afterwards.
Use indexing to hurry up queries. Avoid pointless joins. Normalize or denormalize your info according to your obtain styles. And always monitor database performance as you grow.
In short, the proper database depends upon your app’s construction, speed requirements, and how you expect it to grow. Take time to select sensibly—it’ll help save many issues later on.
Optimize Code and Queries
Rapidly code is vital to scalability. As your app grows, every small hold off provides up. Badly created code or unoptimized queries can decelerate general performance and overload your process. That’s why it’s crucial that you Establish successful logic from the start.
Begin by writing cleanse, basic code. Stay away from repeating logic and remove just about anything unneeded. Don’t choose the most complex Option if a straightforward one particular functions. Keep the features brief, concentrated, and simple to check. Use profiling instruments to discover bottlenecks—places wherever your code can take also long to run or uses an excessive amount memory.
Up coming, evaluate your database queries. These normally sluggish matters down a lot more than the code itself. Be sure Each and every question only asks for the data you truly require. Prevent Choose *, which fetches anything, and rather pick out particular fields. Use indexes to hurry up lookups. And avoid carrying out too many joins, Specially throughout big tables.
In case you notice the identical details becoming requested many times, use caching. Store the outcome quickly using instruments like Redis or Memcached so you don’t must repeat high priced functions.
Also, batch your database operations once you can. In place of updating a row one after the other, update them in teams. This cuts down on overhead and tends to make your app far more successful.
Remember to check with massive datasets. Code and queries that get the job done fine with 100 records may well crash whenever they have to manage one million.
To put it briefly, scalable applications are speedy applications. Keep your code restricted, your queries lean, and use caching when necessary. These methods enable your software keep clean and responsive, at the same time as the load boosts.
Leverage Load Balancing and Caching
As your application grows, it's got to manage additional people and much more site visitors. If every little thing goes by means of a single server, it's going to speedily become a bottleneck. That’s in which load balancing and caching are available in. These two tools assistance keep the application rapidly, secure, and scalable.
Load balancing spreads incoming targeted traffic across several servers. Rather than 1 server doing all the do the job, the load balancer routes people to diverse servers determined by availability. This implies no single server receives overloaded. If one particular server goes down, the load balancer can send out traffic to the Other people. Applications like Nginx, HAProxy, or cloud-dependent answers from AWS and Google Cloud make this easy to arrange.
Caching is about storing facts briefly so it can be reused promptly. When consumers request the exact same data once more—like a product web site or possibly a profile—you don’t have to fetch it within the database every time. You may serve it with the cache.
There are 2 popular forms of caching:
1. Server-aspect caching (like Redis or Memcached) stores facts in memory for rapidly access.
two. Client-aspect caching (like browser caching or CDN caching) suppliers static information near the user.
Caching lessens database load, enhances speed, and can make your application a lot more economical.
Use caching for things that don’t change typically. And always be sure your cache is current when info does transform.
In brief, load balancing and caching are uncomplicated but powerful equipment. Alongside one another, they help your app cope with more consumers, continue to be rapidly, and Get better from issues. If you intend to improve, you need the two.
Use Cloud and Container Instruments
To build scalable applications, you may need instruments that permit your app develop simply. That’s wherever cloud platforms and containers are available. They provide you overall flexibility, lower set up time, and make scaling much smoother.
Cloud platforms like Amazon Internet Providers (AWS), Google Cloud System (GCP), and Microsoft Azure Enable you to hire servers and products and services as you would like them. You don’t have to purchase hardware or guess potential capability. When targeted traffic boosts, you can add much more sources with just a few clicks or immediately utilizing automobile-scaling. When visitors drops, you'll be able to scale down to save money.
These platforms also provide solutions like managed databases, storage, load balancing, and safety resources. You are able to concentrate on building your application in lieu of managing infrastructure.
Containers are another vital Resource. A container deals your app and every thing it needs to operate—code, libraries, options—into a single unit. This can make it effortless to move your app concerning environments, from the laptop to the cloud, with out surprises. Docker is the preferred Resource for this.
When your application works by using several containers, tools like Kubernetes assist you take care of them. Kubernetes handles deployment, scaling, and recovery. If one aspect of one's application crashes, it restarts it routinely.
Containers also allow it to be straightforward to independent parts of your application into solutions. You are able to update or scale pieces independently, that's perfect for functionality and reliability.
Briefly, making use of cloud and container applications implies you can scale rapidly, deploy easily, and Get well quickly when troubles happen. If you need your application to expand without the need of limitations, start employing these applications early. They conserve time, lower danger, and allow you to continue to be focused on constructing, not correcting.
Keep track of Almost everything
If you don’t check your software, you received’t know when things go Improper. Checking allows you see how your app is accomplishing, spot concerns early, and make superior conclusions as your app grows. It’s a important Section of making scalable systems.
Begin by tracking standard metrics like CPU use, memory, disk House, and reaction time. These show you how your servers and services are carrying out. Equipment like Prometheus, Grafana, Datadog, or New Relic may help you accumulate and visualize this details.
Don’t just monitor your servers—keep track of your application way too. Control how much time it will require for buyers to load internet pages, how frequently faults materialize, and where they occur. Logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can help you see what’s happening within your code.
Arrange alerts for vital complications. For example, In the event your reaction time goes earlier mentioned a Restrict or possibly a provider goes down, it is best to get notified promptly. This can help you correct troubles quickly, frequently prior to users even see.
Checking can be helpful when you make changes. For those who deploy a different characteristic and see a spike in faults or slowdowns, you may roll it back before it will cause true harm.
As your application grows, website traffic and details enhance. With out checking, you’ll overlook signs of issues until finally it’s too late. But with the appropriate resources set up, you remain on top of things.
In a nutshell, checking will help you keep your application reliable and scalable. It’s not almost spotting failures—it’s about comprehension your method and making certain it works properly, even under pressure.
Ultimate Thoughts
Scalability isn’t just for large corporations. Developers blog Even little applications need a robust Basis. By developing diligently, optimizing properly, and utilizing the ideal equipment, you could Construct applications that grow easily devoid of breaking under pressure. Commence compact, Believe massive, and Establish wise.